diff --git a/README.md b/README.md
index 5493c7b..41100a2 100644
--- a/README.md
+++ b/README.md
@@ -10,23 +10,28 @@ This is written completely from scratch in C using the [vitaGL library](https://
width="250px"
alt="Screenshot 1"
title="Screenshot 1"
- src="https://github.com/m-bartlett/vita-tetris/assets/85039141/91056539-ab7c-4868-a940-d249f4ff0a6e"
+ src="https://github.com/m-bartlett/vita-tetris/assets/85039141/62095254-35c7-4df2-b1b1-95537dabb9c6"
>
+
+### 🚀 See this project's [FuHEN Homebrew Contest 2023 submission](https://fuhen.homebrew-contest.com/submissions/52/)
+
+
## Disclaimer
This homebrew application is in no way affiliated with The Tetris Company, or any other third parties.
@@ -52,7 +57,7 @@ Please support official Tetris® releases.
Simply download the [latest release .vpk](https://github.com/m-bartlett/vita-tetris/releases/latest/download/ViTetris.vpk) to your Vita and install by your preferred means. You should see a ViTetris bubble on the homescreen.
-
+
@@ -81,3 +86,5 @@ vita-pack-vpk \
```
To build and run in the Vita3K emulator, run `Vita3K` which will be in `$PATH` after running `bootstrap-vita-sdk 3k` and configure it with the firmware and font packages as instructed (you will need to download these from the internet). Once Vita3K is configured with those files, run `make 3k` to either trigger an initial installation of the built `.vpk` file, or update the assets of an existing installation of ViTetris in the emulator.
+
+For a more detailed explanation for what library configuration is necessary to support building for Vita3K, see [Setting Up the Vita SDK to Compile for the Vita3K Emulator](Setting%20Up%20the%20Vita%20SDK%20to%20Compile%20for%20the%20Vita3K%20Emulator.md).
diff --git a/Setting Up the Vita SDK to Compile for the Vita3K Emulator .md b/Setting Up the Vita SDK to Compile for the Vita3K Emulator.md
similarity index 93%
rename from Setting Up the Vita SDK to Compile for the Vita3K Emulator .md
rename to Setting Up the Vita SDK to Compile for the Vita3K Emulator.md
index fd10b77..38b8876 100644
--- a/Setting Up the Vita SDK to Compile for the Vita3K Emulator .md
+++ b/Setting Up the Vita SDK to Compile for the Vita3K Emulator.md
@@ -1,6 +1,6 @@
## Setting up vitaGL with Vita3K Emulator Support
-
+The included script [./sdk/bootstrap-vita-sdk](./sdk/bootstrap-vita-sdk) will handle the below setup steps for you automatically. However the configuration details are explained for any who are curious.
### vitaShaRK (**vita** **Sha**ders **R**untime **K**ompiler)
@@ -66,4 +66,4 @@ It may take up to a minute depending on your machine, but just observe the title
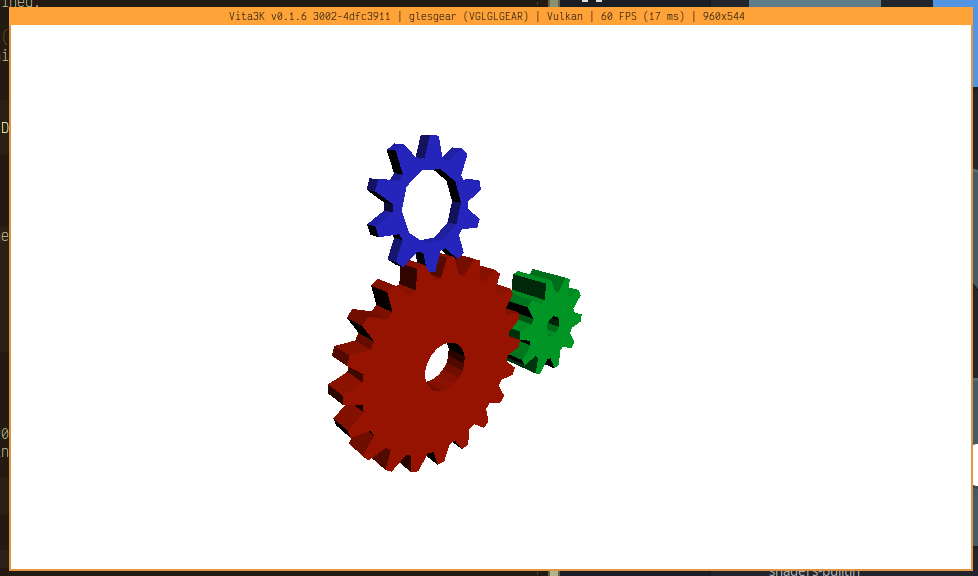
-If Vita3K can run the demo, your $VITASDK environment is correctly setup to support creating executables that are compatible with Vita3K.
+If Vita3K can run the demo, your $VITASDK environment is correctly setup to support creating executables that are compatible with Vita3K.
\ No newline at end of file
diff --git a/src/engine/core.c b/src/engine/core.c
index d62e1f5..3d8bd1e 100644
--- a/src/engine/core.c
+++ b/src/engine/core.c
@@ -66,8 +66,8 @@ static inline void engine_input_callback_analog_right(uint8_t x, uint8_t y) {
enum _ {ANALOG_DEADZONE=10};
float model_matrix[16] = {[0]=1, [5]=1, [10]=1, [15]=1};
float _x=((float)x)-127.f, _y=((float)y)-127.f;
- if (fabs(_x) < ANALOG_DEADZONE) _x = 0.f; else _x = M_PI/8*_x/127.f;
- if (fabs(_y) < ANALOG_DEADZONE) _y = 0.f; else _y = M_PI/8*_y/127.f;
+ if (fabs(_x) < ANALOG_DEADZONE) _x = 0.f; else _x = M_PI/5*_x/127.f;
+ if (fabs(_y) < ANALOG_DEADZONE) _y = 0.f; else _y = M_PI/5*_y/127.f;
translate(model_matrix, PLAYFIELD_WIDTH/2, PLAYFIELD_HEIGHT/2, 0);
rotate(model_matrix, _x, 0, 1, 0);
@@ -116,14 +116,16 @@ void engine_init()
engine_spawn_tetromino(engine_pop_queued_tetromino());
scoring_init();
gravity_timer=timer_get_current_time();
- engine_state = ENGINE_STATE_RUNNING;
- gravity_timer=timer_get_current_time();
/*}}}*/ }
void engine_main_loop(void) {
while(engine_state != ENGINE_STATE_EXIT) {
switch(engine_state) {
+ case ENGINE_STATE_NULL:
+ case ENGINE_STATE_INIT:
+ engine_init();
+ engine_press_start_loop();
case ENGINE_STATE_RUNNING:
engine_game_loop();
break;
@@ -134,12 +136,12 @@ void engine_main_loop(void) {
case ENGINE_STATE_LOSE:
graphics_core_animate_game_over();
sceKernelDelayThread(1500000);
- engine_replay_loop();
+ engine_state = ENGINE_STATE_INIT;
break;
case ENGINE_STATE_WIN:
graphics_core_animate_game_win();
sceKernelDelayThread(1500000);
- engine_replay_loop();
+ engine_state = ENGINE_STATE_INIT;
break;
}
}
@@ -195,7 +197,7 @@ void engine_pause_loop(void) {
}
-void engine_replay_loop(void) {
+void engine_press_start_loop(void) {
SceCtrlData input;
for (int i = 0; i < 4; ++i) { // Update all buffers in rotation, required for input updates
@@ -203,8 +205,7 @@ void engine_replay_loop(void) {
graphics_core_draw_HUD();
const float height = 3;
graphics_text_draw_ad_hoc("PRESS START",-18.5/3.f, height, 2.0);
- graphics_text_draw_ad_hoc("TO", -4/3.f, height-2, 2.0);
- graphics_text_draw_ad_hoc("PLAY AGAIN",-17/3.f, height-4, 2.0);
+ graphics_text_draw_ad_hoc("TO PLAY", -11.5/3.f, height-1.5, 2.0);
sceCtrlPeekBufferPositive(0, &input, 1);
vglSwapBuffers(GL_FALSE);
}
diff --git a/src/engine/core.h b/src/engine/core.h
index 381cf50..b0d66ea 100644
--- a/src/engine/core.h
+++ b/src/engine/core.h
@@ -12,6 +12,7 @@
enum engine_state_enum { ENGINE_STATE_NULL=0,
+ ENGINE_STATE_INIT,
ENGINE_STATE_RUNNING,
ENGINE_STATE_PAUSED,
ENGINE_STATE_LOSE,
@@ -34,7 +35,7 @@ void engine_end();
void engine_main_loop(void);
void engine_game_loop(void);
void engine_pause_loop(void);
-void engine_replay_loop(void);
+void engine_press_start_loop(void);
void engine_spawn_tetromino(tetromino_type_t type);
tetromino_type_t engine_pop_queued_tetromino();
bool engine_move_falling_tetromino(int8_t dx, uint8_t dy);
diff --git a/src/engine/input.c b/src/engine/input.c
index 66fe0ee..b106ca4 100644
--- a/src/engine/input.c
+++ b/src/engine/input.c
@@ -42,8 +42,8 @@ void input_read_and_run_callbacks()
sceCtrlPeekBufferPositive(0, &input, 1);
check_button_single_fire(up)
- check_button_held_repeats(right, /*debounce_microseconds=*/68500)
- check_button_held_repeats(left, /*debounce_microseconds=*/68500)
+ check_button_held_repeats(right, /*debounce_microseconds=*/71000)
+ check_button_held_repeats(left, /*debounce_microseconds=*/71000)
check_button_held_repeats(down, /*debounce_microseconds=*/35000)
check_button_single_fire(triangle)